Simple Contact List with ListTile
Simple Contact List with ListTile
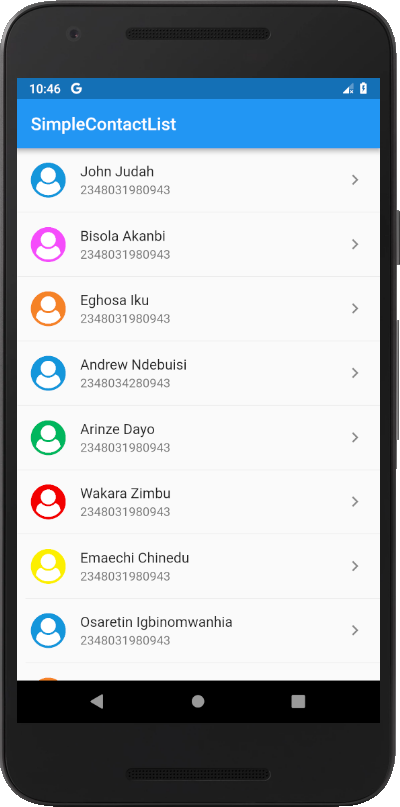
According to the Flutter API reference documentation a ListTile is a single fixed-height row that typically contains some text as well as a leading or trailing icon.
Today, we are going to see how to build a simple Flutter app to enable us practice with the ListTile Class. We’ll call it SimpleContactList. This app will have just one screen which contains Names, Phone numbers, pictures and a right arrow icon as its trailing for each individual arranged as a list.
Add Images to Your Project
Open Android Studio and create a new flutter project. Delete all the code from lib/main.dart. Create a directory called images to store the images. To use these images we must add a few lines of code in our pubspec.yaml. In pubspec add a section named assets directly under uses-material-design. Make sure that these sections line up to avoid running into errors. Once you’re finished hit packages get in the top right corner of the window.
# The following section is specific to Flutter.
flutter:
# The following line ensures that the Material Icons font is
# included with your application, so that you can use the icons in
# the material Icons class.
uses-material-design: true
assets:
- images/avatar.png
- images/avatar_green.png
- images/avatar_red.png
- images/avatar_yellow.png
- images/avatar_purple.png
- images/avatar_brown.png
# To add assets to your application, add an assets section, like this:
# assets:
# - assets.images/a_dot_burr.jpeg
# - assets.images/a_dot_ham.jpeg
Create app in Stateless Widget
In the main.dat file create the main method which is an entry point to the app. We call runApp here. The main() method uses arrow (=>) notation. The app extends a StatelessWidget.
The Scaffold widget, from the Material library, provides a default app bar, title, and a body property that holds the widget tree for the screen. The body consists of a ListView Widget containing children Widget.
class MyApp extends StatelessWidget {
[@override](http://twitter.com/override)
Widget build(BuildContext context) {
final title = 'SimpleContactList';</span><span id="4c0b" class="dz kq jh fq kr b dj kv kw kx ky kz kt s ku">return MaterialApp(
debugShowCheckedModeBanner: false,
title: title,
home: Scaffold(
appBar: AppBar(
title: Text(title),
),
body: ListView(
children: <Widget>[],
),
),
);
}
}</span>
Add ListTile Widget
ListTile(
leading: CircleAvatar(
backgroundColor: Colors.white,
backgroundImage: AssetImage('images/avatar.png'),
),
title: Text(
'John Judah',
),
subtitle: Text('2348031980943'),
trailing: Icon(Icons.keyboard_arrow_right),
onTap: () {
Text('Another data');
},
),</span>
Leading is the widget to display before the title, usually an Icon or a CircleAvatar widget. We used properties like background color and background Image to set color and images that display at the background.
Title is the primary content of the list tile typically a Text widget.
Subtitle is an additional content displayed below the title.
Trailing is a widget to display after the title, typically an Icon widget. We used and _keyboard_arrowright icon.
onTap is called when the user taps this list tile.
Including Divider
new Divider(
height: 1.0,
indent: 1.0,
),</span>
The Divider is a one device pixel thick horizontal line, with padding on either side.
Complete Code
import 'package:flutter/material.dart';
void main() => runApp(MyApp());
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
final title = 'SimpleContactList';
return MaterialApp(
debugShowCheckedModeBanner: false,
title: title,
home: Scaffold(
appBar: AppBar(
title: Text(title),
),
body: ListView(
children: <Widget>[
ListTile(
leading: CircleAvatar(
backgroundColor: Colors.white,
backgroundImage: AssetImage('images/avatar.png'),
),
title: Text(
'John Judah',
),
subtitle: Text('2348031980943'),
trailing: Icon(Icons.keyboard_arrow_right),
onTap: () {
Text('Another data');
},
),
new Divider(
height: 1.0,
indent: 1.0,
),
ListTile(
leading: CircleAvatar(
backgroundColor: Colors.white,
backgroundImage: AssetImage('images/avatar_purple.png'),
),
title: Text('Bisola Akanbi'),
subtitle: Text('2348031980943'),
trailing: Icon(Icons.keyboard_arrow_right),
onTap: () {
Text('Another data');
},
onLongPress: () {
Text('Data');
},
),
new Divider(
height: 1.0,
indent: 1.0,
),
ListTile(
leading: CircleAvatar(
backgroundColor: Colors.white,
backgroundImage: AssetImage('images/avatar_brown.png'),
),
title: Text('Eghosa Iku'),
subtitle: Text('2348031980943'),
trailing: Icon(Icons.keyboard_arrow_right),
),
new Divider(
height: 1.0,
indent: 1.0,
),
ListTile(
leading: CircleAvatar(
backgroundColor: Colors.white,
backgroundImage: AssetImage('images/avatar.png'),
),
title: Text(
'Andrew Ndebuisi',
),
subtitle: Text('2348034280943'),
trailing: Icon(Icons.keyboard_arrow_right),
),
new Divider(
height: 1.0,
indent: 1.0,
),
ListTile(
leading: CircleAvatar(
backgroundColor: Colors.white,
backgroundImage: AssetImage('images/avatar_green.png'),
),
title: Text('Arinze Dayo'),
subtitle: Text('2348031980943'),
trailing: Icon(Icons.keyboard_arrow_right),
),
new Divider(
height: 1.0,
indent: 1.0,
),
ListTile(
leading: CircleAvatar(
backgroundColor: Colors.white,
backgroundImage: AssetImage('images/avatar_red.png'),
),
title: Text('Wakara Zimbu'),
subtitle: Text('2348031980943'),
trailing: Icon(Icons.keyboard_arrow_right),
),
new Divider(
height: 1.0,
indent: 1.0,
),
ListTile(
leading: CircleAvatar(
backgroundColor: Colors.white,
backgroundImage: AssetImage('images/avatar_yellow.png'),
),
title: Text('Emaechi Chinedu'),
subtitle: Text('2348031980943'),
trailing: Icon(Icons.keyboard_arrow_right),
),
new Divider(
height: 1.0,
indent: 10.0,
),
ListTile(
leading: CircleAvatar(
backgroundColor: Colors.white,
backgroundImage: AssetImage('images/avatar.png'),
),
title: Text('Osaretin Igbinomwanhia'),
subtitle: Text('2348031980943'),
trailing: Icon(Icons.keyboard_arrow_right),
),
new Divider(
height: 1.0,
indent: 10.0,
),
ListTile(
leading: CircleAvatar(
backgroundColor: Colors.white,
backgroundImage: AssetImage('images/avatar_brown.png'),
),
title: Text('Osagumwenro Nosa'),
subtitle: Text('2348031980943'),
trailing: Icon(Icons.keyboard_arrow_right),
),
],
),
),
);
}
}